Introduction to Keyban Auth
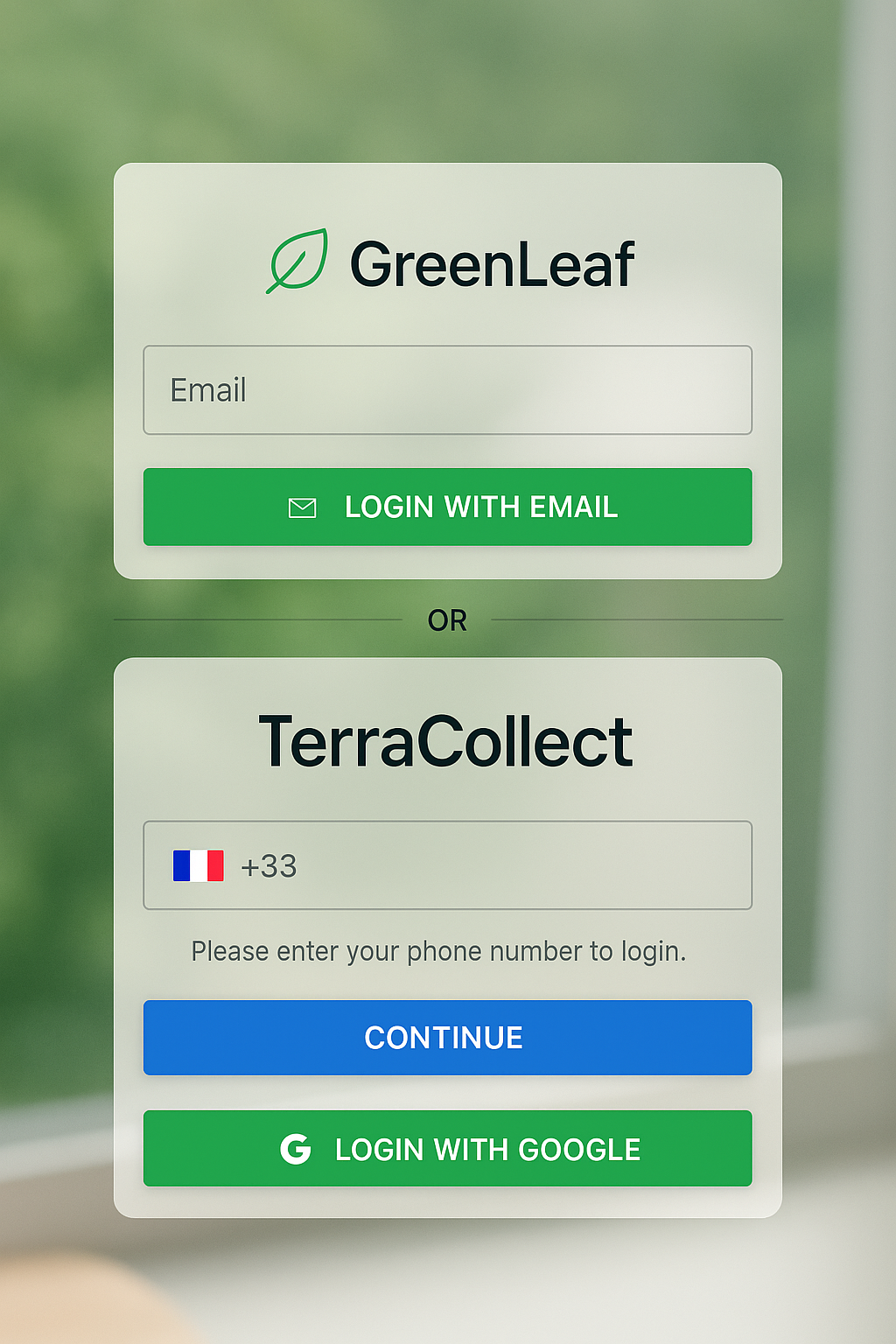
Keyban Auth is a secure and modular authentication layer designed to simplify the way users access non-custodial wallets.
It enables integrators to offer custom login experiences while benefiting from high-security key management and seamless wallet orchestration.
Whether you need a branded login interface, integration with an existing SSO, or support for modern authentication flows (social login, OTP, passkeys), Keyban Auth adapts to your needs with minimal integration effort.
You can fully customize the login interface using the <SignIn />
component, adapting it to your design system and branding.
Developer Entry Points
To implement authentication in your React app, use:
useKeybanAuth
– to manage authentication state, session lifecycle, and access to the wallet.<SignIn />
– a customizable login component that initiates authentication flows and can be adapted to your brand UI.
Both are available via the Keyban SDK for React and compatible with React Native.
Example: Minimal Phone-based Login with OTP
import { useKeybanAuth } from "@keyban/sdk-react";
export default function Login() {
const [phone, setPhone] = useState("");
const [otp, setOtp] = useState("");
const [step, setStep] = useState<"phone" | "otp">("phone");
const { passwordlessStart, passwordlessLogin, isAuthenticated } = useKeybanAuth();
if (isAuthenticated) return <div>You're in!</div>;
return step === "phone" ? (
<form onSubmit={async (e) => {
e.preventDefault();
await passwordlessStart("sms", phone);
setStep("otp");
}}>
<input value={phone} onChange={(e) => setPhone(e.target.value)} />
<button type="submit">Send Code</button>
</form>
) : (
<form onSubmit={async (e) => {
e.preventDefault();
await passwordlessLogin("sms", phone, otp);
}}>
<input value={otp} onChange={(e) => setOtp(e.target.value)} />
<button type="submit">Verify</button>
</form>
);
}
This minimal integration uses useKeybanAuth()
to perform an OTP-based login flow with only a few lines of code. It can be fully customized and styled to match your brand.
Use Cases
Full Auth and Wallet Orchestration via Keyban Auth
Description
In this scenario, Keyban Auth handles both user authentication and wallet management end-to-end. This configuration is particularly suited for non-financial contexts where integrators require a complete solution without managing keys themselves.
The wallets are classified as non-custodial because:
- Client shares: Stored securely using the KeybanStorageProvider, protected by the same advanced security mechanisms as server shares.
- Server shares: Managed by Keyban services, using advanced encryption.
Responsibility Separation
To maximize security:
- Client shares and server shares are stored in two separate databases, reducing risks in the event of a breach.
- Both shares are encrypted using keys protected by HSMs certified to FIPS 140-2 Level 3.
Actions such as balance inquiries, transaction signatures, and DKG (Distributed Key Generation) operations require user authentication, ensuring that only legitimate users can initiate operations.
Diagram
Key Features
- Mandatory Authentication: All interactions with the wallet (balance inquiry, signatures, DKG) require authentication through Keyban Auth.
- Enhanced Security:
- Advanced encryption for both client and server shares.
- Separate storage of shares in different databases to avoid single points of failure.
- Non-Custodial Solution:
While Keyban orchestrates operations such as signing or DKG, the keys remain securely protected and cannot be used without the user’s explicit consent.
Benefits
- High Security:
- Critical data (client and server shares) benefits from robust protection through physical separation and encryption.
- HSM certification ensures compliance with the highest security standards.
- Simplified Compliance: Tailored for integrators in non-financial environments.
- Seamless User Experience: Modern authentication simplifies access while maintaining maximum security.
Integrator-Managed Auth with Keyban Wallet Integration
Description
In this scenario, the integrator uses their proprietary authentication system for the primary application while integrating Keyban Auth for wallet management. This approach allows the integrator to customize the user experience while leveraging Keyban’s advanced features.
- Client shares: Managed by the integrator using a proprietary StorageProvider, secured through the main application’s authentication mechanism.
- Server shares: Managed by Keyban for operations requiring signatures or DKG.
Diagram
Key Features
- Proprietary Authentication: The integrator retains control over access to the main application.
- Flexible Access: Authentication is required only for sensitive operations (DKG, signatures), while balance inquiries remain open.
- Separation of Responsibilities: Keyban Auth integrates seamlessly with existing infrastructure while handling advanced wallet operations.
Benefits
- Integrator Control: User flows are customizable to meet specific needs.
- Optimized User Experience: Authentication is only required for critical operations, reducing friction.
- Evolving Security: Benefits from Keyban Auth’s advanced mechanisms without compromising existing systems.